Notice
Recent Posts
Recent Comments
Link
yusukaid's IT note
Python 병렬 라이브러리 개발 프로젝트 - 코드 분할: pyconnx.py 본문
※본 포스팅은 22년 9월~22년 11에 진행된 프로젝트의 연구노트입니다.
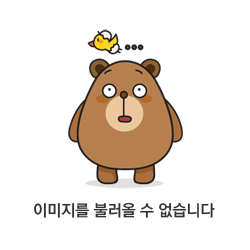
원래는 함수, 구조체별로 코드를 분할 해 총 17개의 파일로 만들었으나, 업체에서 라이브러리를 불러오는 코드가 너무 불필요하게 많이 사용되어 시스템 부하의 문제가 발생할 수 있다는 우려가 들어왔다. 따라서 함수, 구조체별로만 코드를 묶어 분할하는 방향으로 프로젝트를 진행했다.
pyconnx.py
- 로직: C 함수들을 모아 놓은 파이썬 파일
- 파일 'pycoonx'로 통합한 함수 목록
- compare()
- alloc()
- convert_input_file()
- convert_output_file()
- init_model_name()
- init()
- load_Model()
- Model_destroy()
- run_Model()
- set_input_count()
import ctypes
import sys
from pyconnx_Types import Tensor
from pyconnx_Types import Model
path =f'./libconnx.so'
connx = ctypes.cdll.LoadLibrary(path)
args = sys.argv
#compare
def compare():
compare = connx.compare
compare.argtypes = [ctypes.POINTER(ctypes.POINTER(Tensor)), ctypes.POINTER(ctypes.POINTER(Tensor))]
compare.restype = ctypes.c_int32
return compare
#connx_alloc
def alloc():
connx_alloc = connx.connx_alloc
connx_alloc.argtypes = [ctypes.c_size_t]
connx_alloc.restype = ctypes.c_void_p
return connx_alloc
#connx_convert_input_file
def convert_input_file():
connx_convert_input_file = connx.connx_convert_input_file
connx_convert_input_file.argtypes = [ctypes.POINTER(ctypes.POINTER(Tensor))]
connx_convert_input_file.restype = ctypes.c_int32
return connx_convert_input_file
#connx_convert_output_file
def convert_output_file():
connx_convert_output_file = connx.connx_convert_output_file
connx_convert_output_file.argtypes = [ctypes.POINTER(ctypes.POINTER(Tensor))]
connx_convert_output_file.restype = ctypes.c_int32
return connx_convert_output_file
#connx_init_model_name
def init_model_name():
connx_init_model_name = connx.connx_init_model_name
connx_init_model_name.argtypes = [ctypes.POINTER(ctypes.c_char)]
connx_init_model_name.restype = ctypes.c_int32
return connx_init_model_name
#connx_init
def init():
connx_init = connx.connx_init
connx_init.argtypes = None
connx_init.restype = None
return connx_init
#connx_load_model
def load_Model():
connx_load_Model = connx.connx_load_Model
connx_load_Model.argtypes = [ctypes.POINTER(Model), ctypes.c_uint32]
connx_load_Model.restype = ctypes.c_int32
return connx_load_Model
#connx_destroy_model
def Model_destroy():
connx_Model_destroy = connx.connx_Model_destroy
connx_Model_destroy.argtypes = [ctypes.POINTER(Model)]
connx_Model_destroy.restype = None
return connx_Model_destroy
#connx_run_model
def run_Model():
connx_run_Model = connx.connx_run_Model
connx_run_Model.argtypes = [ctypes.POINTER(Model), ctypes.POINTER(ctypes.POINTER(Tensor)), ctypes.POINTER(ctypes.POINTER(Tensor))]
connx_run_Model.restype = ctypes.c_int32
return connx_run_Model
#set_input_count
def set_input_count():
temp_set_input_count = connx.temp_set_input
temp_set_input_count.argtypes = [ctypes.c_uint32]
temp_set_input_count.restype = None
return temp_set_input_count
'python' 카테고리의 다른 글
Python 병렬 라이브러리 개발 프로젝트 - 코드 분할: pyconnx_test.py (0) | 2023.01.12 |
---|---|
Python 병렬 라이브러리 개발 프로젝트 - 코드 분할: pyconnx_Types.py (0) | 2023.01.12 |
Python 병렬 라이브러리 개발 프로젝트 - 순환 참조 오류 (0) | 2023.01.12 |
Python 병렬 라이브러리 개발 프로젝트 - demo 코드 분석 (0) | 2023.01.05 |
Python 병렬 라이브러리 개발 프로젝트 - 프로젝트 구성 (0) | 2023.01.05 |